4.1. Introduction¶
By convention, API documentation for Java code is written directly in the source code in special comments called Javadoc comments. The Javadoc tool is used to parse the source code and Javadoc comments to produce a nicely formatted website for the documentation. Here is an example:
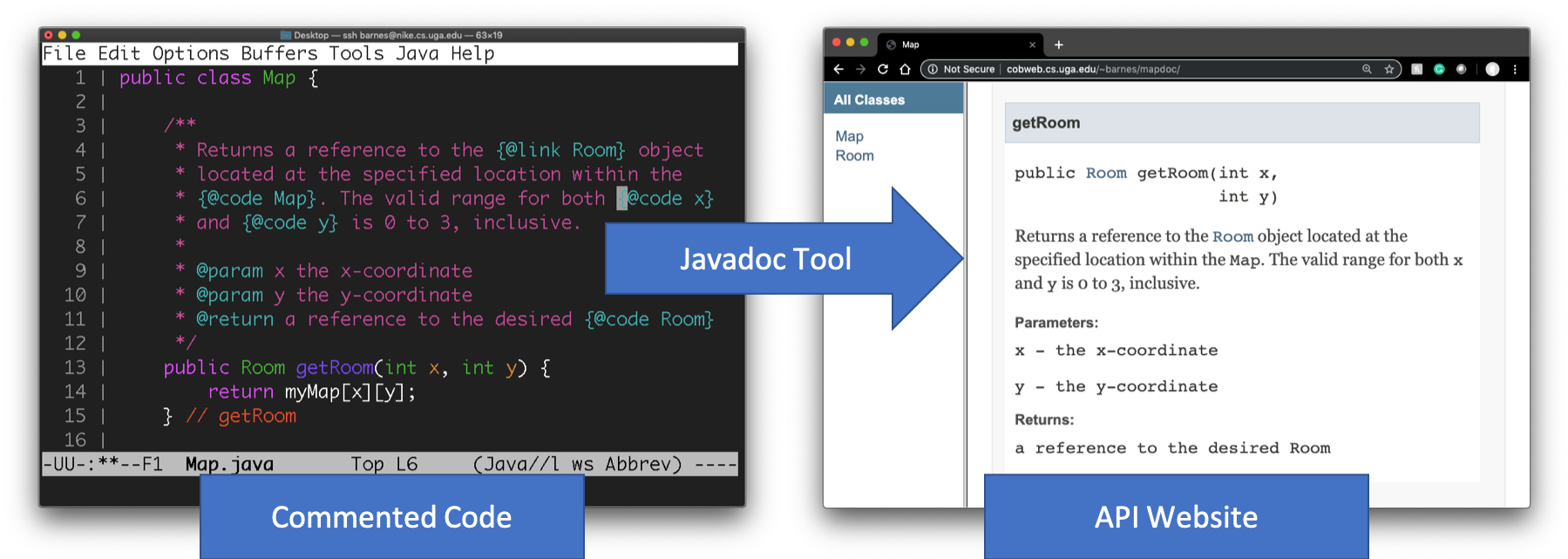
In the figure above, we see a Javadoc comment for the getRoom
method. It starts with /**
and ends with */
. The body of the
comment is a set of correctly punctuated English sentences. Special
@
tags are used throughout the comment to provide semantic context
for terms. Semantic information is used to influence formatting in the
generated website. Toward the end of the comment, you see that the
parameters and the return value are documented using two @param
tags
and a @return
tag.
In this chapter, you will see Javadoc comments in action, write your own, and learn how to generate and host the corresponding API documentation website on Odin.
But first, a few definitions:
Application Programming Interface (API)
A well-written, organized collection of code provides an Application Programming Interface (API) for users of that code. That is, the design choices that were made in the naming of certain things such as classes and methods impose an interface that programmers must follow when interacting with that code.
For example, you’ve undoubtedly used the String class in a program.
If you follow the link to the Java API documentation for String
and
look at the “Method Summary” section, you will see the list of methods
that are available in the String
class. These methods provide an
interface for you, the programmer to interact with the class. The
charAt
method has the following signature:
char charAt(int index)
This tells us that the method expects to receive an int
parameter
and it returns a char
. Also, since we don’t see the keyword
static
in the signature, we know it is an instance method that
should be called using a reference to a valid String
object.
This interface tells us everything we need to know to be able to call
the method.
API Documentation
Knowing how to call the method and what the method does (its semantics) are two separate things. To know what the method does, we cannot (and often don’t want to) look at the code within the method (its implementation). As users of a particular class, we must rely solely on documentation to help determine how that code should be used.
Such documentation is usually referred to as API documentation, and it should be written to provide enough detail so that others can use the code being documented without seeing the implementation of the code itself. In other words, API documentation usually describes what a piece of code does and not how it does what it does (i.e., unless absolutely needed to explain the “what”).
Rapid Fire Review
What is the primary purpose of Javadoc comments in Java code?
To add debugging information
To write special instructions for the compiler
To document the code and generate API documentation
To test the code directly within the comments
What symbol is used to start a Javadoc comment in Java?
//
/*
/**
#
What is the difference between the API and API documentation?
The API refers to the interface programmers must follow to interact with the code, while the API documentation describes the semantics of the code.
The API refers to the user interface, while the API documentation is the implementation of the code.
The API is written in plain English, while the API documentation is written in code.
The API is used to test the code, while the API documentation is used to compile the code.
Why might it be important for API documentation to describe what code does rather than how it does it?
To keep the documentation brief and free of unnecessary details.
To allow users to modify the implementation of the code.
To ensure that users can use the code without needing to understand its internal workings.
To make it easier to debug the code.